nana::gui::treebox
Description
a treebox is a widget that displays a hierarchical list of items, such as the files
and directories on a disk.
Model
of
Widget Window
Public base classes
class widget_object<widget_tag, DrawerTrigger>:
public
widget
Typedefs
item_proxy |
A type refers to the item and also used to iterate through the node. |
ext_event_type |
The type of extra event defined by treebox.(See Note 2) |
node_image_type |
The type of state image. |
renderer_interface |
The interface of treebox item renderer. |
compset_placer_interface |
The interface of treebox compset_placer. |
Members
treebox(); |
The default constructor without creating the widget. |
treebox(window wd, bool visible) |
Creates a treebox in a specified window. |
treebox(window wd, const rectangle& r = rectangle(), bool visible = true) |
Creates a treebox with a specified rectangle. |
void auto_draw(bool) |
Enables/disables the widget that draws automatically when it is operated. |
treebox& checkable(bool enable) |
Enables/disables the checkbox for each item of the widgets. |
bool checkable() const |
Determine whether the checkbox is enabled. |
item_proxy erase(item_proxy pos) |
Erases the item pointed to by pos. |
void erase(const nana::string& keypath) |
Erases the item specified by keypath. |
ext_event_type& ext_event() const |
Retrieves the object of extra events. |
treebox& icon(const nana::string& id, const node_image_type&) |
Sets the state image with a specified id name. |
node_image_type& icon(const nana::string& id) const |
Retrieves the object of state image by the specified id name. |
void icon_erase(const nana::string& id) |
Erases the state image by the specified id name. |
item_proxy find(const nana::string& keypath) |
Find a item though a specified keypath. |
item_proxy insert(const nana::string& keypath, const nana::string& title); |
Inserts a new node to treebox, keypath specifies the node hierarchical, title is
used for displaying. If the keypath is existing, it returns the existing node. |
item_proxy insert(item_proxy pos, const nana::string& key, const nana::string&
title); |
Inserts a new node to treebox, pos is the parent, key specifies the new node, title
is used for displaying. If the key is existing, it returns the existing node. |
nana::string make_key_path(item_proxy pos, const nana::string& splitter); |
It returns the key path of item pointed to by pos, the splitter splits the each owner of node. |
template<typename Placer> treebox& placer(const Placer&) |
Sets a new compset placer. |
const nana::pat::cloneable<compset_placer_interface>& placer() const |
Gets the compset placer. |
template<typename ItemRenderer> treebox& renderer(const ItemRenderer&) |
Sets a new item renderer. |
const nana::pat::cloneable<renderer_interface> & renderer() const |
Gets the item renderer. |
item_proxy selected() const |
Gets the item that is selected. |
Item Proxy (on the concept of Input Iterator)
item_proxy() |
The default constructor creates an end iterator. |
item_proxy append(const nana::string& key, const nana::string& name) |
Append a child. |
template<typename T>
item_proxy append(const nana::string& key, const nana::string& name, const T& usr_obj) |
Append a child with a specified user object. |
bool empty() const |
Returns true if the proxy does not refer to a node, as an end iterator. |
std::size_t level() const |
Returns the distance between the ROOT node and this node, the return is only available when empty() is false. |
bool checked() const |
Returns the check state. |
item_proxy& check(bool) |
Sets the check state, and it returns itself. |
bool expanded() const |
Returns true when the node is expanded. |
item_proxy& expand(bool) |
Expand/Shrink children of the node, and it returns itself. |
bool selected() const |
Returns true when the node is selected. |
item_proxy& select(bool) |
Select the node, and it returns itself. |
const nana::string& icon() const |
Returns the id of icon. |
item_proxy& icon(const nana::string& id) |
Set the id of icon. |
const nana::string& text() const |
Returns the text. |
item_proxy& text(const nana::string&); |
Set a new text. |
const nana::string& key() const |
Returns the key. |
item_proxy& key(const nana::string&) |
Set a new key. |
std::size_t size() const |
Returns the number of child nodes. |
item_proxy child() const |
Returns the first child of the node. |
item_proxy owner() const |
Returns the owner of the node. |
item_proxy sibling() const |
Returns the sibling of the node. |
item_proxy begin() const |
Returns an proxy pointing to the beginning of node. |
item_proxy end() const |
Returns an proxy pointing to the end of node. |
bool operator==(const nana::string& s) const |
Compare the text of node with the s. |
bool operator==(const char* s) const |
Ditto. |
bool operator==(const wchar_t* s) const |
Ditto. |
File
nana/gui/widgets/treebox.hpp
Tutorial
Loading the directories into the treebox.
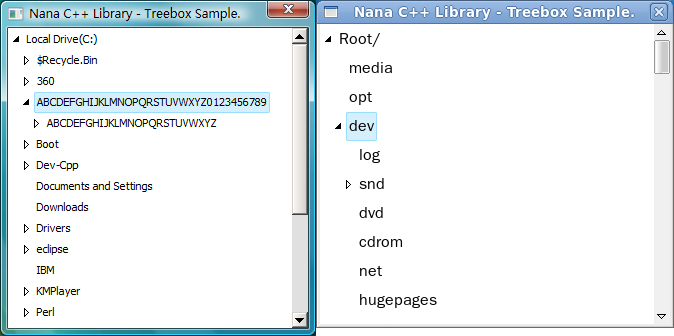
#include <nana/gui/wvl.hpp>
#include <nana/gui/widgets/treebox.hpp>
#include <nana/filesystem/file_iterator.hpp>
class folder_tree
: public nana::gui::form
{
public:
typedef nana::gui::treebox::item_proxy item_proxy;
folder_tree()
:
nana::gui::form(nana::gui::API::make_center(300, 300),
appear::decorate<appear::taskbar>())
{
tree_.create(*this, 0,
0, 300, 300);
#if defined(NANA_WINDOWS)
item_proxy node =
tree_.insert(STR("C:"),
STR("Local Drive(C:)"));
nana::filesystem::file_iterator i(STR("C:\\")),
end;
#elif defined(NANA_LINUX)
//Use a whitespace for the root key since the root under linux
//is / character.
item_proxy node =
tree_.insert(STR("/"),
STR("Root"));
nana::filesystem::file_iterator i(STR("/")),
end;
#endif
for(; i != end; ++i)
{
if(false == i->directory)
continue;
tree_.insert(node, i->name, i->name);
break;
}
tree_.ext_event().expand = nana::make_fun(*this,
&folder_tree::_m_expand);
}
private:
void _m_expand(nana::gui::window, item_proxy node, bool exp)
{
if(!exp) return; //If this is contracted.
//Windows supports the path separator '/'
nana::string path = tree_.make_key_path(node, STR("/")) + STR("/");
//Trim the whitespace at head of the path. So the code can be work
//well in both Windows and Linux.
nana::string::size_type path_start_pos = path.find_first_not_of(STR(" "));
if(path_start_pos != path.npos)
path.erase(0, path_start_pos);
//Walk in the path directory
for sub directories.
nana::filesystem::file_iterator i(path), end;
for(; i != end; ++i)
{
if(false
== i->directory) continue; //If it
is not a directory.
item_proxy child = tree_.insert(node,
i->name, i->name);
if(child.empty()) continue;
//Find a directory
in child directory, if there is a directory,
//insert
it into the child, just insert one node to indicate the
//node has a child
and an arrow symbol will be displayed in the
//front of the node.
nana::filesystem::file_iterator u(path
+ i->name);
for(; u != end; ++u)
{
if(false
== u->directory) continue;
//If it
is not a directory.
tree_.insert(child,
u->name, u->name);
break;
}
}
}
private:
nana::gui::treebox tree_;
};
int main()
{
folder_tree fdtree;
fdtree.show();
nana::gui::exec();
}
Notes
1. The key string is case sensitive.
2. treebox::ext_event_type. Nana.GUI event is a general-purpose, such
as mouse_down, mouse_up, mouse_move event. But some widgets need some events
that satisfies its own specifical demand, so the widget class provides an
ext_event_type for this demand. Its definition is:
struct ext_event_type
{
typedef Implementation-Specified item_proxy;
nana::fn_group<void(nana::gui::window, item_proxy, bool exp)>
expand; //Expands or shrinks an item, exp is true if it is expanded.
nana::fn_group<void(nana::gui::window, item_proxy, bool set)> checked; //The item is checked, set is true if it is checked.
nana::fn_group<void(nana::gui::window, item_proxy, bool set)>
selected; //Selects an item, set is true if it is selected.
};
If a node is expanded or
shrinked, the expand method will be called, and the 3rd
parameter specifies whether the node is expanded.
If a node is selected or unselected, the selected method will be called, and the
3rd parameter specifies whether the node is selected.
3. The definition of node_image_type
struct node_image_type
{
nana::paint::image normal;
nana::paint::image highlighted;
nana::paint::image expanded;
};
See also
None.
Move to The Nana Programmer's Guide Main Page |